Using try and catch in Java…
Example : TryDemo
import java.io.*;
class TryDemo
{
public static void main(String args[])
{
int d=0;
int a;
try
{
a=42/d;
System.out.println("This will not be printed");
}
catch(ArithmeticException e)
{
System.out.println("Division by Zero");
}
System.out.println("After catch statement");
}
}
import java.io.*;
class TryDemo
{
public static void main(String args[])
{
int d=0;
int a;
try
{
a=42/d;
System.out.println("This will not be printed");
}
catch(ArithmeticException e)
{
System.out.println("Division by Zero");
}
System.out.println("After catch statement");
}
}
import java.io.*; class TryDemo { public static void main(String args[]) { int d=0; int a; try { a=42/d; System.out.println("This will not be printed"); } catch(ArithmeticException e) { System.out.println("Division by Zero"); } System.out.println("After catch statement"); } }

Multiple catch clauses in Java…
Example : MultiCatch
import java.io.*;
class MultiCatch
{
public static void main(String args[])
{
try
{
int a=args.length;
System.out.println("a="+a);
int b=42/a;
int c[]={1};
c[42]=99;
}
catch(ArithmeticException e)
{
System.out.println("Exception Occurred:"+e);
}
catch(ArrayIndexOutOfBoundsException e)
{
System.out.println("Exception Occured:"+e);
}
System.out.println("After try/catch blocks.");
}
}
import java.io.*;
class MultiCatch
{
public static void main(String args[])
{
try
{
int a=args.length;
System.out.println("a="+a);
int b=42/a;
int c[]={1};
c[42]=99;
}
catch(ArithmeticException e)
{
System.out.println("Exception Occurred:"+e);
}
catch(ArrayIndexOutOfBoundsException e)
{
System.out.println("Exception Occured:"+e);
}
System.out.println("After try/catch blocks.");
}
}
import java.io.*; class MultiCatch { public static void main(String args[]) { try { int a=args.length; System.out.println("a="+a); int b=42/a; int c[]={1}; c[42]=99; } catch(ArithmeticException e) { System.out.println("Exception Occurred:"+e); } catch(ArrayIndexOutOfBoundsException e) { System.out.println("Exception Occured:"+e); } System.out.println("After try/catch blocks."); } }

Throw in Java…
Example : ThrowDemo
import java.io.*;
class ThrowDemo
{
public static void main(String[] args) throws Exception
{
int number=20;
try
{
if(number >10)
{
throw new Exception("Maximum Limit is 10");
}
else
{
number=Integer.parseInt(args[0]);
}
}
catch(Exception e)
{
System.out.println("Exception has been Occured"+e.toString());
}
finally
{
System.out.println("This is the Last Statement");
}
}
}
import java.io.*;
class ThrowDemo
{
public static void main(String[] args) throws Exception
{
int number=20;
try
{
if(number >10)
{
throw new Exception("Maximum Limit is 10");
}
else
{
number=Integer.parseInt(args[0]);
}
}
catch(Exception e)
{
System.out.println("Exception has been Occured"+e.toString());
}
finally
{
System.out.println("This is the Last Statement");
}
}
}
import java.io.*; class ThrowDemo { public static void main(String[] args) throws Exception { int number=20; try { if(number >10) { throw new Exception("Maximum Limit is 10"); } else { number=Integer.parseInt(args[0]); } } catch(Exception e) { System.out.println("Exception has been Occured"+e.toString()); } finally { System.out.println("This is the Last Statement"); } } }

Finally in Java…
Example : FinallyDemo
import java.io.*;
class FinallyDemo
{
static void procA()
{
try
{
System.out.println("inside procA");
throw new RuntimeException("demo");
}finally
{
System.out.println("proA's finally");
}
}
static void procB()
{
try
{
System.out.println("inside procB");
return;
}finally
{
System.out.println("procB's finally");
}
}
static void procC()
{
try
{
System.out.println("inside procC");
}finally
{
System.out.println("proC's finally");
}
}
public static void main(String args[])
{
try
{
procA();
}catch(Exception e)
{
System.out.println("Exception caught");
}
procB();
procC();
}
}
import java.io.*;
class FinallyDemo
{
static void procA()
{
try
{
System.out.println("inside procA");
throw new RuntimeException("demo");
}finally
{
System.out.println("proA's finally");
}
}
static void procB()
{
try
{
System.out.println("inside procB");
return;
}finally
{
System.out.println("procB's finally");
}
}
static void procC()
{
try
{
System.out.println("inside procC");
}finally
{
System.out.println("proC's finally");
}
}
public static void main(String args[])
{
try
{
procA();
}catch(Exception e)
{
System.out.println("Exception caught");
}
procB();
procC();
}
}
import java.io.*; class FinallyDemo { static void procA() { try { System.out.println("inside procA"); throw new RuntimeException("demo"); }finally { System.out.println("proA's finally"); } } static void procB() { try { System.out.println("inside procB"); return; }finally { System.out.println("procB's finally"); } } static void procC() { try { System.out.println("inside procC"); }finally { System.out.println("proC's finally"); } } public static void main(String args[]) { try { procA(); }catch(Exception e) { System.out.println("Exception caught"); } procB(); procC(); } }
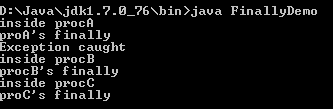