Reader and Writer Classes
FileReader in Java…
Example : FileReaderDemo
import java.io.*;
class FileReaderDemo
{
public static void main(String args[]) throws Exception
{
FileReader fr=new FileReader("FileReaderDemo.java");
BufferedReader br=new BufferedReader(fr);
String s;
while((s=br.readLine()) !=null)
{
System.out.println(s);
}
fr.close();
}
}
import java.io.*;
class FileReaderDemo
{
public static void main(String args[]) throws Exception
{
FileReader fr=new FileReader("FileReaderDemo.java");
BufferedReader br=new BufferedReader(fr);
String s;
while((s=br.readLine()) !=null)
{
System.out.println(s);
}
fr.close();
}
}
import java.io.*; class FileReaderDemo { public static void main(String args[]) throws Exception { FileReader fr=new FileReader("FileReaderDemo.java"); BufferedReader br=new BufferedReader(fr); String s; while((s=br.readLine()) !=null) { System.out.println(s); } fr.close(); } }
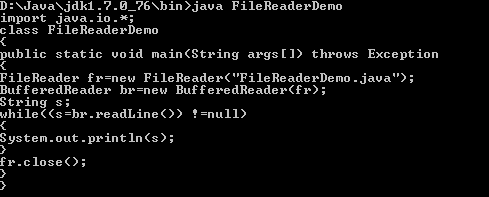
FileWriter in Java…
Example : FileWriterDemo
import java.io.*;
class FileWriterDemo{
public static void main(String args[])throws Exception {
String source="Now is the time for all good men\n"
+"to come to the aid of their country\n"
+"and pay their due taxes.";
char buffer[]=new char[source.length()];
source.getChars(0,source.length(),buffer,0);
FileWriter f1=new FileWriter("file1.txt");
for (int i=0;i<buffer.length;i+=2) {
f1.write(buffer[i]);
}
f1.close();
FileWriter f2 = new FileWriter("file2.txt");
f2.write(buffer);
f2.close();
FileWriter f3 = new FileWriter("file3.txt");
f3.write(buffer,buffer.length-buffer.length/4,buffer.length/4);
f3.close();
}
}
import java.io.*;
class FileWriterDemo{
public static void main(String args[])throws Exception {
String source="Now is the time for all good men\n"
+"to come to the aid of their country\n"
+"and pay their due taxes.";
char buffer[]=new char[source.length()];
source.getChars(0,source.length(),buffer,0);
FileWriter f1=new FileWriter("file1.txt");
for (int i=0;i<buffer.length;i+=2) {
f1.write(buffer[i]);
}
f1.close();
FileWriter f2 = new FileWriter("file2.txt");
f2.write(buffer);
f2.close();
FileWriter f3 = new FileWriter("file3.txt");
f3.write(buffer,buffer.length-buffer.length/4,buffer.length/4);
f3.close();
}
}
import java.io.*; class FileWriterDemo{ public static void main(String args[])throws Exception { String source="Now is the time for all good men\n" +"to come to the aid of their country\n" +"and pay their due taxes."; char buffer[]=new char[source.length()]; source.getChars(0,source.length(),buffer,0); FileWriter f1=new FileWriter("file1.txt"); for (int i=0;i<buffer.length;i+=2) { f1.write(buffer[i]); } f1.close(); FileWriter f2 = new FileWriter("file2.txt"); f2.write(buffer); f2.close(); FileWriter f3 = new FileWriter("file3.txt"); f3.write(buffer,buffer.length-buffer.length/4,buffer.length/4); f3.close(); } }

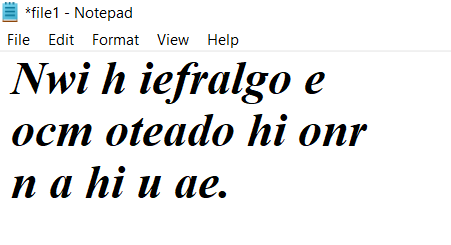
Output : File1
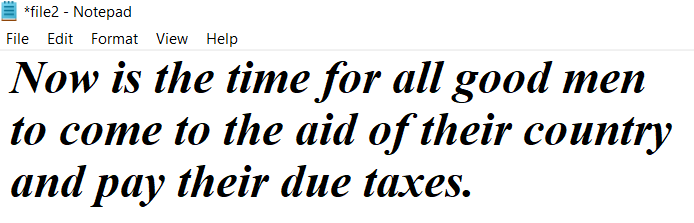
Output : File2

Output : File3
CharArrayReader in Java…
Example : CharArrayReaderDemo
import java.io.*;
public class CharArrayReaderDemo
{
public static void main(String args[])throws IOException
{
String temp="abcdefghijklmnopqrstvwxyz";
int length=temp.length();
char c[]=new char[length];
temp.getChars(0,length,c,0);
CharArrayReader input1=new CharArrayReader(c);
CharArrayReader input2=new CharArrayReader(c,0,5);
int I;
System.out.println("input1 is:");
while((I=input1.read())!=-1)
{
System.out.print((char)I);
}
System.out.println();
System.out.println("input2 is:");
while((I=input2.read())!=-1)
{
System.out.print((char)I);
}
System.out.println();
}
}
import java.io.*;
public class CharArrayReaderDemo
{
public static void main(String args[])throws IOException
{
String temp="abcdefghijklmnopqrstvwxyz";
int length=temp.length();
char c[]=new char[length];
temp.getChars(0,length,c,0);
CharArrayReader input1=new CharArrayReader(c);
CharArrayReader input2=new CharArrayReader(c,0,5);
int I;
System.out.println("input1 is:");
while((I=input1.read())!=-1)
{
System.out.print((char)I);
}
System.out.println();
System.out.println("input2 is:");
while((I=input2.read())!=-1)
{
System.out.print((char)I);
}
System.out.println();
}
}
import java.io.*; public class CharArrayReaderDemo { public static void main(String args[])throws IOException { String temp="abcdefghijklmnopqrstvwxyz"; int length=temp.length(); char c[]=new char[length]; temp.getChars(0,length,c,0); CharArrayReader input1=new CharArrayReader(c); CharArrayReader input2=new CharArrayReader(c,0,5); int I; System.out.println("input1 is:"); while((I=input1.read())!=-1) { System.out.print((char)I); } System.out.println(); System.out.println("input2 is:"); while((I=input2.read())!=-1) { System.out.print((char)I); } System.out.println(); } }

CharArrayWriter in Java…
Example : CharArrayWriterDemo
import java.io.*;
class CharArrayWriterDemo
{
public static void main(String args[])throws IOException
{
CharArrayWriter f=new CharArrayWriter();
String s="The should end up in the array";
char buf[]=new char[s.length()];
s.getChars(0,s.length(),buf,0);
f.write(buf);
System.out.println("Buffer as a string");
System.out.println(f.toString());
System.out.println("Into array");
char c[]=f.toCharArray();
for(int i=0;i<c.length;i++)
{
System.out.print(c[i]);
}
System.out.println("\nTo FileWriter()");
FileWriter f2=new FileWriter("test.txt");
f.writeTo(f2);
f2.close();
System.out.println("Doing a reset");
f.reset();
for(int i=0;i<3;i++)
f.write('X');
System.out.println(f.toString());
}
}
import java.io.*;
class CharArrayWriterDemo
{
public static void main(String args[])throws IOException
{
CharArrayWriter f=new CharArrayWriter();
String s="The should end up in the array";
char buf[]=new char[s.length()];
s.getChars(0,s.length(),buf,0);
f.write(buf);
System.out.println("Buffer as a string");
System.out.println(f.toString());
System.out.println("Into array");
char c[]=f.toCharArray();
for(int i=0;i<c.length;i++)
{
System.out.print(c[i]);
}
System.out.println("\nTo FileWriter()");
FileWriter f2=new FileWriter("test.txt");
f.writeTo(f2);
f2.close();
System.out.println("Doing a reset");
f.reset();
for(int i=0;i<3;i++)
f.write('X');
System.out.println(f.toString());
}
}
import java.io.*; class CharArrayWriterDemo { public static void main(String args[])throws IOException { CharArrayWriter f=new CharArrayWriter(); String s="The should end up in the array"; char buf[]=new char[s.length()]; s.getChars(0,s.length(),buf,0); f.write(buf); System.out.println("Buffer as a string"); System.out.println(f.toString()); System.out.println("Into array"); char c[]=f.toCharArray(); for(int i=0;i<c.length;i++) { System.out.print(c[i]); } System.out.println("\nTo FileWriter()"); FileWriter f2=new FileWriter("test.txt"); f.writeTo(f2); f2.close(); System.out.println("Doing a reset"); f.reset(); for(int i=0;i<3;i++) f.write('X'); System.out.println(f.toString()); } }

BufferedReader in Java…
Example : BRReadLines
import java.io.*;
class BRReadLines
{
public static void main(String args[]) throws IOException
{
BufferedReader br=new BufferedReader(new InputStreamReader(System.in));
String str;
System.out.println("Enter lines of text.");
System.out.println("Enter 'stop' to quit.");
do
{
str=br.readLine();
System.out.println(str);
}
while(!str.equals("stop"));
}
}
import java.io.*;
class BRReadLines
{
public static void main(String args[]) throws IOException
{
BufferedReader br=new BufferedReader(new InputStreamReader(System.in));
String str;
System.out.println("Enter lines of text.");
System.out.println("Enter 'stop' to quit.");
do
{
str=br.readLine();
System.out.println(str);
}
while(!str.equals("stop"));
}
}
import java.io.*; class BRReadLines { public static void main(String args[]) throws IOException { BufferedReader br=new BufferedReader(new InputStreamReader(System.in)); String str; System.out.println("Enter lines of text."); System.out.println("Enter 'stop' to quit."); do { str=br.readLine(); System.out.println(str); } while(!str.equals("stop")); } }
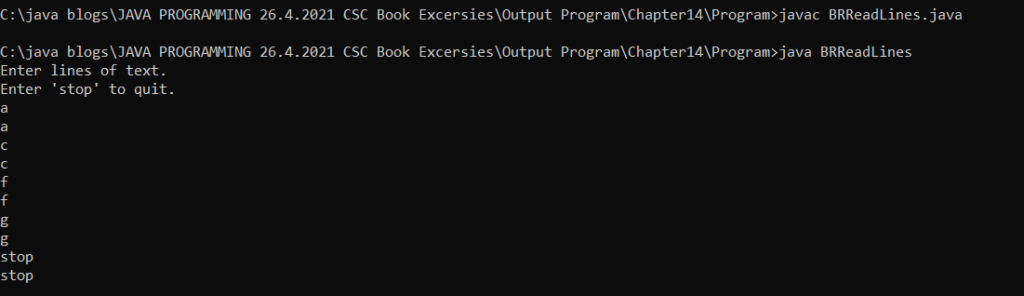
BufferedWriter in Java…
Example : PrintWriterDemo
import java.io.*;
public class PrintWriterDemo
{
public static void main(String args[])
{
PrintWriter pw=new PrintWriter(System.out,true);
pw.println("This is a string");
int i=-7;
pw.println(i);
double d=4.5e-7;
pw.println(d);
}
}
import java.io.*;
public class PrintWriterDemo
{
public static void main(String args[])
{
PrintWriter pw=new PrintWriter(System.out,true);
pw.println("This is a string");
int i=-7;
pw.println(i);
double d=4.5e-7;
pw.println(d);
}
}
import java.io.*; public class PrintWriterDemo { public static void main(String args[]) { PrintWriter pw=new PrintWriter(System.out,true); pw.println("This is a string"); int i=-7; pw.println(i); double d=4.5e-7; pw.println(d); } }
