Calling Methods…
Example : BoxDemo
import java.io.*; class Box { double width; double height; double depth; } class BoxDemo{ public static void main(String args[]) { Box mybox=new Box(); double vol; mybox.width=10; mybox.height=20; mybox.depth=15; vol=mybox.width*mybox.height*mybox.depth; System.out.println("volume is "+vol); } }

Overloading methods…
Example : OverloadDemo
import java.io.*; class OverloadDemo { void multi() { System.out.println("No value to multiply"); } void multi(int a) { System.out.println("a*a:"+a*a); } void multi(int a,int b) { System.out.println(a+"and "+b+":"+a*b); } double multi(double a) { return a*a; } public static void main(String args[]) { OverloadDemo ob=new OverloadDemo(); double result; ob.multi(); ob.multi(10); ob.multi(10,20); result=ob.multi(123.25); System.out.println("Result of ob.multi(123.25):"+result); } }
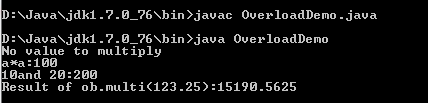
Argument passing in java…
Call-by-Value…
import java.io.*; class CallTest { void change(int i) { i=i+1; System.out.println("Inside function i="+i); } } class callbyvalue { public static void main(String[] args) { CallTest ob=new CallTest(); int a=10; System.out.println("Before call a="+a); ob.change(a); System.out.println("After call a="+a); } }

Call-by-Reference…
import java.io.*; class RefTest { int a,b; void change(RefTest o) { o.a+=2; o.b-=2; } void show() { System.out.println("A="+a+",B="+b); } } class CallByRef { public static void main(String args[]) { RefTest ob1=new RefTest(); RefTest ob2=new RefTest(); ob2.a=10; ob2.b=20; System.out.println("Before calling change"); ob2.show(); ob1.change(ob2); System.out.println("After calling change"); ob2.show(); } }
