Flow Layout in Java…
Example : FlowLayoutDemo
import java.applet.*;
import java.awt.*;
/*
<applet code="FlowLayoutDemo" width=300 height=200>
</applet>
*/
public class FlowLayoutDemo extends Applet
{
public void init()
{
setLayout(new FlowLayout(FlowLayout.CENTER,5,5));
for(int i=1;i<=10;i++)
{
add(new Button("BUTTON "+i));
}
}
}
import java.applet.*;
import java.awt.*;
/*
<applet code="FlowLayoutDemo" width=300 height=200>
</applet>
*/
public class FlowLayoutDemo extends Applet
{
public void init()
{
setLayout(new FlowLayout(FlowLayout.CENTER,5,5));
for(int i=1;i<=10;i++)
{
add(new Button("BUTTON "+i));
}
}
}
import java.applet.*; import java.awt.*; /* <applet code="FlowLayoutDemo" width=300 height=200> </applet> */ public class FlowLayoutDemo extends Applet { public void init() { setLayout(new FlowLayout(FlowLayout.CENTER,5,5)); for(int i=1;i<=10;i++) { add(new Button("BUTTON "+i)); } } }

Grid Layout…
Example : GridLayoutDemo
import java.awt.*;
import java.applet.*;
/*
<applet code="GridLayoutDemo" width=300 height=200>
</applet>
*/public class GridLayoutDemo extends Applet
{
static final int n=4;
public void init()
{
setLayout(new GridLayout(n,n));
setFont(new Font("SansSerif",Font.BOLD,24));
for(int i=0;i<n;i++)
for(int j=0;j<n;j++)
{
{
int k=i*n+j;
if(k>0)
add(new Button(""+k));
}
}
}
}
import java.awt.*;
import java.applet.*;
/*
<applet code="GridLayoutDemo" width=300 height=200>
</applet>
*/public class GridLayoutDemo extends Applet
{
static final int n=4;
public void init()
{
setLayout(new GridLayout(n,n));
setFont(new Font("SansSerif",Font.BOLD,24));
for(int i=0;i<n;i++)
for(int j=0;j<n;j++)
{
{
int k=i*n+j;
if(k>0)
add(new Button(""+k));
}
}
}
}
import java.awt.*; import java.applet.*; /* <applet code="GridLayoutDemo" width=300 height=200> </applet> */public class GridLayoutDemo extends Applet { static final int n=4; public void init() { setLayout(new GridLayout(n,n)); setFont(new Font("SansSerif",Font.BOLD,24)); for(int i=0;i<n;i++) for(int j=0;j<n;j++) { { int k=i*n+j; if(k>0) add(new Button(""+k)); } } } }
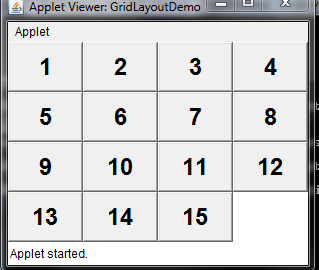
Border Layouts…
Example : BorderLayoutDemo
import java.awt.*;
import java.applet.*;
import java.util.*;
/*
<applet code="BorderLayoutDemo" width=400 height=200>
</applet>
*/
public class BorderLayoutDemo extends Applet
{
public void init()
{
setLayout(new BorderLayout());
add(new Button("This is across the top."),BorderLayout.NORTH);
add(new Label("The Footer message might go here."),BorderLayout.SOUTH);
add(new Button("Right"),BorderLayout.EAST);
add(new Button("Left"),BorderLayout.WEST);
String msg="The reason able man addas"+ "him self to the world;\n"+"the unreasonable onw presits in"+"trying to adopts the world to himself.\n"+"Therefre all progream depends"+"On the unreasonalbe man.\n\n"+"-George Berand shaw\n\n";
add(new TextArea(msg),BorderLayout.CENTER);
}
}
import java.awt.*;
import java.applet.*;
import java.util.*;
/*
<applet code="BorderLayoutDemo" width=400 height=200>
</applet>
*/
public class BorderLayoutDemo extends Applet
{
public void init()
{
setLayout(new BorderLayout());
add(new Button("This is across the top."),BorderLayout.NORTH);
add(new Label("The Footer message might go here."),BorderLayout.SOUTH);
add(new Button("Right"),BorderLayout.EAST);
add(new Button("Left"),BorderLayout.WEST);
String msg="The reason able man addas"+ "him self to the world;\n"+"the unreasonable onw presits in"+"trying to adopts the world to himself.\n"+"Therefre all progream depends"+"On the unreasonalbe man.\n\n"+"-George Berand shaw\n\n";
add(new TextArea(msg),BorderLayout.CENTER);
}
}
import java.awt.*; import java.applet.*; import java.util.*; /* <applet code="BorderLayoutDemo" width=400 height=200> </applet> */ public class BorderLayoutDemo extends Applet { public void init() { setLayout(new BorderLayout()); add(new Button("This is across the top."),BorderLayout.NORTH); add(new Label("The Footer message might go here."),BorderLayout.SOUTH); add(new Button("Right"),BorderLayout.EAST); add(new Button("Left"),BorderLayout.WEST); String msg="The reason able man addas"+ "him self to the world;\n"+"the unreasonable onw presits in"+"trying to adopts the world to himself.\n"+"Therefre all progream depends"+"On the unreasonalbe man.\n\n"+"-George Berand shaw\n\n"; add(new TextArea(msg),BorderLayout.CENTER); } }

Insets…
Example : BorderText
import java.awt.*;
/*
<applet code="BorderText" width=200 height=300>
</applet>
*/
public class BorderText extends java.applet.Applet
{
public void init()
{
setLayout(new BorderLayout(5,5));
add("South",new Button("Button of the Applet"));
add("North",new Button("Top of the Applet"));
add("East",new Button("Right"));
add("West",new Button("Left"));
add("Center",new TextArea("Appears at the center"));
}
public Insets getInsets()
{
return new Insets(20,20,10,10);
}
}
import java.awt.*;
/*
<applet code="BorderText" width=200 height=300>
</applet>
*/
public class BorderText extends java.applet.Applet
{
public void init()
{
setLayout(new BorderLayout(5,5));
add("South",new Button("Button of the Applet"));
add("North",new Button("Top of the Applet"));
add("East",new Button("Right"));
add("West",new Button("Left"));
add("Center",new TextArea("Appears at the center"));
}
public Insets getInsets()
{
return new Insets(20,20,10,10);
}
}
import java.awt.*; /* <applet code="BorderText" width=200 height=300> </applet> */ public class BorderText extends java.applet.Applet { public void init() { setLayout(new BorderLayout(5,5)); add("South",new Button("Button of the Applet")); add("North",new Button("Top of the Applet")); add("East",new Button("Right")); add("West",new Button("Left")); add("Center",new TextArea("Appears at the center")); } public Insets getInsets() { return new Insets(20,20,10,10); } }
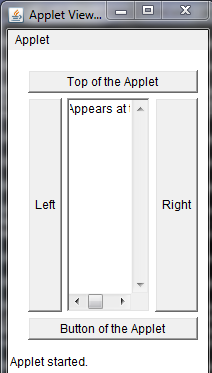
Creating a Frame Window in an Applet…
Example : FrameApplet
import java.applet.*;
import java.awt.*;
import java.awt.event.*;
/*
<applet code="FrameApplet.class"width=250 height=200>
</applet>
*/
class Frame2 extends Frame
{
Frame2(String title)
{
super(title);
addWindowListener(new WindowAdapter(){
public void windowClosing(WindowEvent we)
{
dispose();
}
});
}
}
public class FrameApplet extends Applet implements ActionListener
{
public void init()
{
Button b=new Button("Create Frame");
b.addActionListener(this);
add(b);
}
public void actionPerformed(ActionEvent ae)
{
Frame2 f2=new Frame2("My new Window");
f2.show();
f2.setSize(300,300);
}
}
import java.applet.*;
import java.awt.*;
import java.awt.event.*;
/*
<applet code="FrameApplet.class"width=250 height=200>
</applet>
*/
class Frame2 extends Frame
{
Frame2(String title)
{
super(title);
addWindowListener(new WindowAdapter(){
public void windowClosing(WindowEvent we)
{
dispose();
}
});
}
}
public class FrameApplet extends Applet implements ActionListener
{
public void init()
{
Button b=new Button("Create Frame");
b.addActionListener(this);
add(b);
}
public void actionPerformed(ActionEvent ae)
{
Frame2 f2=new Frame2("My new Window");
f2.show();
f2.setSize(300,300);
}
}
import java.applet.*; import java.awt.*; import java.awt.event.*; /* <applet code="FrameApplet.class"width=250 height=200> </applet> */ class Frame2 extends Frame { Frame2(String title) { super(title); addWindowListener(new WindowAdapter(){ public void windowClosing(WindowEvent we) { dispose(); } }); } } public class FrameApplet extends Applet implements ActionListener { public void init() { Button b=new Button("Create Frame"); b.addActionListener(this); add(b); } public void actionPerformed(ActionEvent ae) { Frame2 f2=new Frame2("My new Window"); f2.show(); f2.setSize(300,300); } }

Menu Actions…
Example : MenuDemo
import java.awt.*;
import java.awt.event.*;
import java.applet.*;
/*
<applet code="MenuDemo"width=250 height=250>
</applet>
*/
class MenuFrame extends Frame
{
String msg="";
CheckboxMenuItem debug,test;
MenuFrame(String title)
{
super(title);
MenuBar mbar=new MenuBar();
setMenuBar(mbar);
Menu file=new Menu("File");
MenuItem item1,item2,item3,item4,item5;
file.add(item1=new MenuItem("New"));
file.add(item2=new MenuItem("Open"));
file.add(item3=new MenuItem("Close"));
file.add(item4=new MenuItem("-"));
file.add(item5=new MenuItem("Quit"));
mbar.add(file);
Menu edit=new Menu("Edit");
MenuItem item6,item7,item8,item9;
edit.add(item6=new MenuItem("Cut"));
edit.add(item7=new MenuItem("Copy"));
edit.add(item8=new MenuItem("Paste"));
edit.add(item9=new MenuItem("-"));
Menu sub=new Menu("Special");
MenuItem item10,item11,item12;
sub.add(item10=new MenuItem("First"));
sub.add(item11=new MenuItem("Second"));
sub.add(item12=new MenuItem("Third"));
edit.add(sub);
debug=new CheckboxMenuItem("Debug");
edit.add(debug);
test=new CheckboxMenuItem("Testing");
edit.add(test);
mbar.add(edit);
MyMenuHandler handler=new MyMenuHandler(this);
item1.addActionListener(handler);
item2.addActionListener(handler);
item3.addActionListener(handler);
item4.addActionListener(handler);
item5.addActionListener(handler);
item6.addActionListener(handler);
item7.addActionListener(handler);
item8.addActionListener(handler);
item9.addActionListener(handler);
item10.addActionListener(handler);
item11.addActionListener(handler);
item12.addActionListener(handler);
debug.addItemListener(handler);
test.addItemListener(handler);
MyWindowAdapter adapter=new MyWindowAdapter(this);
addWindowListener(adapter);
}
public void paint(Graphics g)
{
g.drawString(msg,10,200);
if(debug.getState())
g.drawString("Debug is on.",10,220);
else
g.drawString("Debug is off.",10,220);
if(test.getState())
g.drawString("Testing is on.",10,240);
else
g.drawString("Testing is off.",10,240);
}
}
class MyWindowAdapter extends WindowAdapter
{
MenuFrame menuFrame;
public MyWindowAdapter(MenuFrame menuFrame)
{
this.menuFrame=menuFrame;
}
public void WindowClosing(WindowEvent we)
{
menuFrame.setVisible(false);
}
}
class MyMenuHandler implements ActionListener,ItemListener
{
MenuFrame menuFrame;
public MyMenuHandler(MenuFrame menuFrame)
{
this.menuFrame=menuFrame;
}
public void actionPerformed(ActionEvent ae)
{
String msg="You selected";
String arg=(String)ae.getActionCommand();
if(arg.equals("New"))
msg+="New.";
else if(arg.equals("Open"))
msg+="Open.";
else if(arg.equals("Close"))
msg+="Close.";
else if(arg.equals("Quit"))
msg+="Quit.";
else if(arg.equals("Edit"))
msg+="Edit.";
else if(arg.equals("Cut"))
msg+="Cut.";
else if(arg.equals("Copy"))
msg+="Copy.";
else if(arg.equals("Paste"))
msg+="Paste.";
else if(arg.equals("First"))
msg+="First.";
else if(arg.equals("Second"))
msg+="Second.";
else if(arg.equals("Third"))
msg+="Third.";
else if(arg.equals("Debug"))
msg+="Debug.";
else if(arg.equals("Testing"))
msg+="Testing.";
menuFrame.msg=msg;
menuFrame.repaint();
}
public void itemStateChanged(ItemEvent ie)
{
menuFrame.repaint();
}
}
public class MenuDemo extends Applet
{
Frame f;
public void init()
{
f=new MenuFrame("MenuDemo");
int width=Integer.parseInt(getParameter("width"));
int height=Integer.parseInt(getParameter("height"));
setSize(new Dimension(width,height));
f.setSize(width,height);
f.setVisible(true);
}
public void start()
{
f.setVisible(true);
}
public void stop()
{
f.setVisible(false);
}
}
import java.awt.*;
import java.awt.event.*;
import java.applet.*;
/*
<applet code="MenuDemo"width=250 height=250>
</applet>
*/
class MenuFrame extends Frame
{
String msg="";
CheckboxMenuItem debug,test;
MenuFrame(String title)
{
super(title);
MenuBar mbar=new MenuBar();
setMenuBar(mbar);
Menu file=new Menu("File");
MenuItem item1,item2,item3,item4,item5;
file.add(item1=new MenuItem("New"));
file.add(item2=new MenuItem("Open"));
file.add(item3=new MenuItem("Close"));
file.add(item4=new MenuItem("-"));
file.add(item5=new MenuItem("Quit"));
mbar.add(file);
Menu edit=new Menu("Edit");
MenuItem item6,item7,item8,item9;
edit.add(item6=new MenuItem("Cut"));
edit.add(item7=new MenuItem("Copy"));
edit.add(item8=new MenuItem("Paste"));
edit.add(item9=new MenuItem("-"));
Menu sub=new Menu("Special");
MenuItem item10,item11,item12;
sub.add(item10=new MenuItem("First"));
sub.add(item11=new MenuItem("Second"));
sub.add(item12=new MenuItem("Third"));
edit.add(sub);
debug=new CheckboxMenuItem("Debug");
edit.add(debug);
test=new CheckboxMenuItem("Testing");
edit.add(test);
mbar.add(edit);
MyMenuHandler handler=new MyMenuHandler(this);
item1.addActionListener(handler);
item2.addActionListener(handler);
item3.addActionListener(handler);
item4.addActionListener(handler);
item5.addActionListener(handler);
item6.addActionListener(handler);
item7.addActionListener(handler);
item8.addActionListener(handler);
item9.addActionListener(handler);
item10.addActionListener(handler);
item11.addActionListener(handler);
item12.addActionListener(handler);
debug.addItemListener(handler);
test.addItemListener(handler);
MyWindowAdapter adapter=new MyWindowAdapter(this);
addWindowListener(adapter);
}
public void paint(Graphics g)
{
g.drawString(msg,10,200);
if(debug.getState())
g.drawString("Debug is on.",10,220);
else
g.drawString("Debug is off.",10,220);
if(test.getState())
g.drawString("Testing is on.",10,240);
else
g.drawString("Testing is off.",10,240);
}
}
class MyWindowAdapter extends WindowAdapter
{
MenuFrame menuFrame;
public MyWindowAdapter(MenuFrame menuFrame)
{
this.menuFrame=menuFrame;
}
public void WindowClosing(WindowEvent we)
{
menuFrame.setVisible(false);
}
}
class MyMenuHandler implements ActionListener,ItemListener
{
MenuFrame menuFrame;
public MyMenuHandler(MenuFrame menuFrame)
{
this.menuFrame=menuFrame;
}
public void actionPerformed(ActionEvent ae)
{
String msg="You selected";
String arg=(String)ae.getActionCommand();
if(arg.equals("New"))
msg+="New.";
else if(arg.equals("Open"))
msg+="Open.";
else if(arg.equals("Close"))
msg+="Close.";
else if(arg.equals("Quit"))
msg+="Quit.";
else if(arg.equals("Edit"))
msg+="Edit.";
else if(arg.equals("Cut"))
msg+="Cut.";
else if(arg.equals("Copy"))
msg+="Copy.";
else if(arg.equals("Paste"))
msg+="Paste.";
else if(arg.equals("First"))
msg+="First.";
else if(arg.equals("Second"))
msg+="Second.";
else if(arg.equals("Third"))
msg+="Third.";
else if(arg.equals("Debug"))
msg+="Debug.";
else if(arg.equals("Testing"))
msg+="Testing.";
menuFrame.msg=msg;
menuFrame.repaint();
}
public void itemStateChanged(ItemEvent ie)
{
menuFrame.repaint();
}
}
public class MenuDemo extends Applet
{
Frame f;
public void init()
{
f=new MenuFrame("MenuDemo");
int width=Integer.parseInt(getParameter("width"));
int height=Integer.parseInt(getParameter("height"));
setSize(new Dimension(width,height));
f.setSize(width,height);
f.setVisible(true);
}
public void start()
{
f.setVisible(true);
}
public void stop()
{
f.setVisible(false);
}
}
import java.awt.*; import java.awt.event.*; import java.applet.*; /* <applet code="MenuDemo"width=250 height=250> </applet> */ class MenuFrame extends Frame { String msg=""; CheckboxMenuItem debug,test; MenuFrame(String title) { super(title); MenuBar mbar=new MenuBar(); setMenuBar(mbar); Menu file=new Menu("File"); MenuItem item1,item2,item3,item4,item5; file.add(item1=new MenuItem("New")); file.add(item2=new MenuItem("Open")); file.add(item3=new MenuItem("Close")); file.add(item4=new MenuItem("-")); file.add(item5=new MenuItem("Quit")); mbar.add(file); Menu edit=new Menu("Edit"); MenuItem item6,item7,item8,item9; edit.add(item6=new MenuItem("Cut")); edit.add(item7=new MenuItem("Copy")); edit.add(item8=new MenuItem("Paste")); edit.add(item9=new MenuItem("-")); Menu sub=new Menu("Special"); MenuItem item10,item11,item12; sub.add(item10=new MenuItem("First")); sub.add(item11=new MenuItem("Second")); sub.add(item12=new MenuItem("Third")); edit.add(sub); debug=new CheckboxMenuItem("Debug"); edit.add(debug); test=new CheckboxMenuItem("Testing"); edit.add(test); mbar.add(edit); MyMenuHandler handler=new MyMenuHandler(this); item1.addActionListener(handler); item2.addActionListener(handler); item3.addActionListener(handler); item4.addActionListener(handler); item5.addActionListener(handler); item6.addActionListener(handler); item7.addActionListener(handler); item8.addActionListener(handler); item9.addActionListener(handler); item10.addActionListener(handler); item11.addActionListener(handler); item12.addActionListener(handler); debug.addItemListener(handler); test.addItemListener(handler); MyWindowAdapter adapter=new MyWindowAdapter(this); addWindowListener(adapter); } public void paint(Graphics g) { g.drawString(msg,10,200); if(debug.getState()) g.drawString("Debug is on.",10,220); else g.drawString("Debug is off.",10,220); if(test.getState()) g.drawString("Testing is on.",10,240); else g.drawString("Testing is off.",10,240); } } class MyWindowAdapter extends WindowAdapter { MenuFrame menuFrame; public MyWindowAdapter(MenuFrame menuFrame) { this.menuFrame=menuFrame; } public void WindowClosing(WindowEvent we) { menuFrame.setVisible(false); } } class MyMenuHandler implements ActionListener,ItemListener { MenuFrame menuFrame; public MyMenuHandler(MenuFrame menuFrame) { this.menuFrame=menuFrame; } public void actionPerformed(ActionEvent ae) { String msg="You selected"; String arg=(String)ae.getActionCommand(); if(arg.equals("New")) msg+="New."; else if(arg.equals("Open")) msg+="Open."; else if(arg.equals("Close")) msg+="Close."; else if(arg.equals("Quit")) msg+="Quit."; else if(arg.equals("Edit")) msg+="Edit."; else if(arg.equals("Cut")) msg+="Cut."; else if(arg.equals("Copy")) msg+="Copy."; else if(arg.equals("Paste")) msg+="Paste."; else if(arg.equals("First")) msg+="First."; else if(arg.equals("Second")) msg+="Second."; else if(arg.equals("Third")) msg+="Third."; else if(arg.equals("Debug")) msg+="Debug."; else if(arg.equals("Testing")) msg+="Testing."; menuFrame.msg=msg; menuFrame.repaint(); } public void itemStateChanged(ItemEvent ie) { menuFrame.repaint(); } } public class MenuDemo extends Applet { Frame f; public void init() { f=new MenuFrame("MenuDemo"); int width=Integer.parseInt(getParameter("width")); int height=Integer.parseInt(getParameter("height")); setSize(new Dimension(width,height)); f.setSize(width,height); f.setVisible(true); } public void start() { f.setVisible(true); } public void stop() { f.setVisible(false); } }
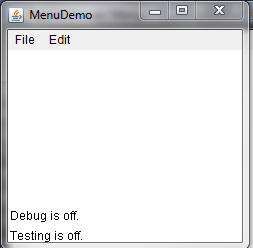
Dialog Boxes..
Example : MessageBox
import java.awt.*;
import java.awt.event.*;
class MessageBox extends Dialog
{
MessageBox(Frame fm,String lab)
{
super(fm,"Message",true);
setLayout(new GridLayout(2,1,0,0));
Panel p1=new Panel();
Panel p2=new Panel();
Button b1,b2;
p1.setFont(new Font("TimesRoman",Font.BOLD,18));
p1.setLayout(new FlowLayout(FlowLayout.CENTER,20,15));
p2.setLayout(new FlowLayout(FlowLayout.CENTER,20,20));
p1.add(new Label(lab));
b1=new Button("ok");
b1.addActionListener(new B1());
p2.add(b1);
b2=new Button("cancel");
b2.addActionListener(new B1());
p2.add(b2);
add(p1);
add(p2);
setSize(350,125);
setTitle("MessageBox");
addWindowListener(new WindowAdapter()
{
public void windowClosing(WindowEvent w)
{
System.exit(0);
}
});
}
class B1 implements ActionListener
{
public void actionPerformed(ActionEvent e)
{
try
{
Button ok=(Button)e.getSource();
String s=ok.getLabel();
if(s.equals("ok")||s.equals("cancel"))
{
dispose();
System.exit(0);
}
}
catch(Exception n){}
}
}
}
//----------MessageApplication Clss-----------
public class MessageApplication extends Frame
{
boolean a;
MessageApplication()
{
MessageBox mb=new MessageBox(this,"JavaAlert:This is a Message Box");
mb.setLocation(200,200);
mb.setVisible(true);
a=false;
}
public static void main(String args[])
{
MessageApplication fm=new MessageApplication();
System.out.println("popping out message box");
fm.setVisible(true);
}
}
import java.awt.*;
import java.awt.event.*;
class MessageBox extends Dialog
{
MessageBox(Frame fm,String lab)
{
super(fm,"Message",true);
setLayout(new GridLayout(2,1,0,0));
Panel p1=new Panel();
Panel p2=new Panel();
Button b1,b2;
p1.setFont(new Font("TimesRoman",Font.BOLD,18));
p1.setLayout(new FlowLayout(FlowLayout.CENTER,20,15));
p2.setLayout(new FlowLayout(FlowLayout.CENTER,20,20));
p1.add(new Label(lab));
b1=new Button("ok");
b1.addActionListener(new B1());
p2.add(b1);
b2=new Button("cancel");
b2.addActionListener(new B1());
p2.add(b2);
add(p1);
add(p2);
setSize(350,125);
setTitle("MessageBox");
addWindowListener(new WindowAdapter()
{
public void windowClosing(WindowEvent w)
{
System.exit(0);
}
});
}
class B1 implements ActionListener
{
public void actionPerformed(ActionEvent e)
{
try
{
Button ok=(Button)e.getSource();
String s=ok.getLabel();
if(s.equals("ok")||s.equals("cancel"))
{
dispose();
System.exit(0);
}
}
catch(Exception n){}
}
}
}
//----------MessageApplication Clss-----------
public class MessageApplication extends Frame
{
boolean a;
MessageApplication()
{
MessageBox mb=new MessageBox(this,"JavaAlert:This is a Message Box");
mb.setLocation(200,200);
mb.setVisible(true);
a=false;
}
public static void main(String args[])
{
MessageApplication fm=new MessageApplication();
System.out.println("popping out message box");
fm.setVisible(true);
}
}
import java.awt.*; import java.awt.event.*; class MessageBox extends Dialog { MessageBox(Frame fm,String lab) { super(fm,"Message",true); setLayout(new GridLayout(2,1,0,0)); Panel p1=new Panel(); Panel p2=new Panel(); Button b1,b2; p1.setFont(new Font("TimesRoman",Font.BOLD,18)); p1.setLayout(new FlowLayout(FlowLayout.CENTER,20,15)); p2.setLayout(new FlowLayout(FlowLayout.CENTER,20,20)); p1.add(new Label(lab)); b1=new Button("ok"); b1.addActionListener(new B1()); p2.add(b1); b2=new Button("cancel"); b2.addActionListener(new B1()); p2.add(b2); add(p1); add(p2); setSize(350,125); setTitle("MessageBox"); addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent w) { System.exit(0); } }); } class B1 implements ActionListener { public void actionPerformed(ActionEvent e) { try { Button ok=(Button)e.getSource(); String s=ok.getLabel(); if(s.equals("ok")||s.equals("cancel")) { dispose(); System.exit(0); } } catch(Exception n){} } } } //----------MessageApplication Clss----------- public class MessageApplication extends Frame { boolean a; MessageApplication() { MessageBox mb=new MessageBox(this,"JavaAlert:This is a Message Box"); mb.setLocation(200,200); mb.setVisible(true); a=false; } public static void main(String args[]) { MessageApplication fm=new MessageApplication(); System.out.println("popping out message box"); fm.setVisible(true); } }
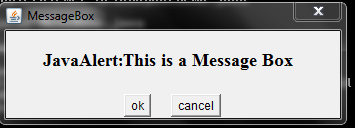
File Dialogs…
Example : FileDialogDemo
import java.awt.*;
import java.awt.event.*;
class FileDialogDemo extends Frame implements ActionListener
{
Frame t;
Button b1;
Label l1;
FileDialogDemo()
{
t=new Frame("Sample Frame");
t.setLayout(new FlowLayout());
b1=new Button("Open");
l1=new Label("File selected:");
t.add(b1);
t.add(l1);
b1.addActionListener(this);
t.setSize(300,300);
t.setVisible(true);
}
public static void main(String args[])
{
FileDialogDemo fd=new FileDialogDemo();
}
public void actionPerformed(ActionEvent a)
{
FileDialog fd=new FileDialog(t,"FileDialog");
fd.setVisible(true);
l1.setText(l1.getText()+fd.getFile());
}
}
import java.awt.*;
import java.awt.event.*;
class FileDialogDemo extends Frame implements ActionListener
{
Frame t;
Button b1;
Label l1;
FileDialogDemo()
{
t=new Frame("Sample Frame");
t.setLayout(new FlowLayout());
b1=new Button("Open");
l1=new Label("File selected:");
t.add(b1);
t.add(l1);
b1.addActionListener(this);
t.setSize(300,300);
t.setVisible(true);
}
public static void main(String args[])
{
FileDialogDemo fd=new FileDialogDemo();
}
public void actionPerformed(ActionEvent a)
{
FileDialog fd=new FileDialog(t,"FileDialog");
fd.setVisible(true);
l1.setText(l1.getText()+fd.getFile());
}
}
import java.awt.*; import java.awt.event.*; class FileDialogDemo extends Frame implements ActionListener { Frame t; Button b1; Label l1; FileDialogDemo() { t=new Frame("Sample Frame"); t.setLayout(new FlowLayout()); b1=new Button("Open"); l1=new Label("File selected:"); t.add(b1); t.add(l1); b1.addActionListener(this); t.setSize(300,300); t.setVisible(true); } public static void main(String args[]) { FileDialogDemo fd=new FileDialogDemo(); } public void actionPerformed(ActionEvent a) { FileDialog fd=new FileDialog(t,"FileDialog"); fd.setVisible(true); l1.setText(l1.getText()+fd.getFile()); } }
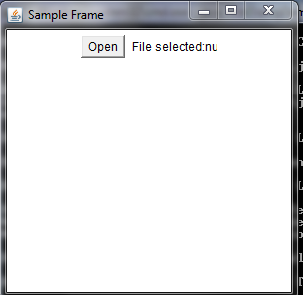