1.Comment Statement :
This statement is not for compiler or Interpreter but for another Human only.This helps in making the program more easily readable.
Uses :
-Comments can be used to explain Python code.
-Comments can be used to make the code more readable.
-Comments can be used to prevent execution when testing code.
Multi Line Comments: – source code
""" this is a comment written in more than just one line""" print("Hello, student!")
Multi Line Comments: – output

2.Declaration Statement:
There is no statement for declaration.The language does not require declaring variables before its usage,like in other language. Pyhton does not use compiler for memory allocation for variables.Hence declaring the variable is not needed.
3.Assignment Statement:
Example 1: Assingment statement – source code
a,b,c,d=5,"kumar",10+2j,500.45 print(a) print(b) print(c) print(d)
Example 1: Assingment statement – Output

4.Input statement and Output statement:
Input: It means any information or data sent to the computer from the user through the keyboard or other devices is called input Output: It means the information produced by the computer to the user is called output
The syntax for input function is
input (prompt_message)
Example 2: Input Function-source code
a= eval(input("Give a number")) print("value is ",a,"and the object data class is",type(a))
Example 2: Input Function-Output

How to take inputs for the Sequence Data Types like List, Set, Tuple, etc.
Formatting Output:
Example 3:Python String formatting using F string – source code
# Initialising a variable name = "ABC" # Output print(f'Hello{name}!How are you?')
Example 3:Python String formatting using F string – Output

Using Format()
We can also use the format() function, to format output to make it looks Presentable. The curly braces {} works as placeholders. We can specify the order in which variables occur in the output.
Using implicit position:– source code
n = input("What is your Name") en = input("Give the Enrolment Number") print("The Default Order output\n") print("Hai, {}, Welcome to Python Strings Class and your Enrolment Number is {}".format(n, en))
Using implicit position:– Output
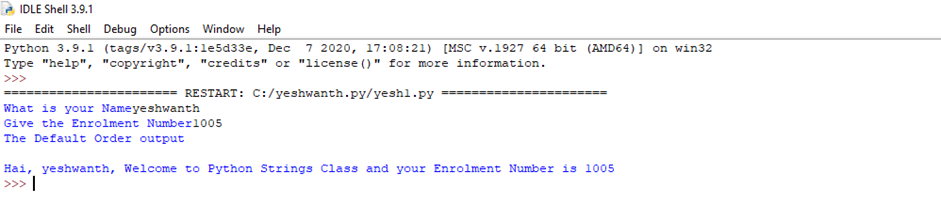
Using Position order:-source code
n = input("What is your Name") en = input("Give the Enrolment Number") print("The Position Order output\n") print("Hai, {1}, Welcome to Python Strings Class and your Enrolment number is {0}".format(en, n))
Using Position order:-Output
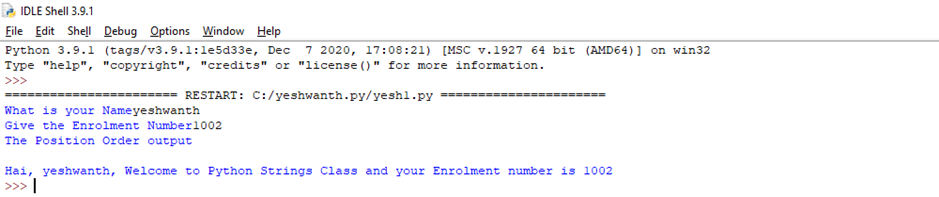
Using Keyboard Order:-Source code
n = input("What is Your Name") en = input("Give the Enrolment Number") print("The Keyword Order output\n") print("Hai, {n}, Welcome to Python Strings Class and your Enrollment Number is{en}".format(n=15,en=15))
Using Keyboard Order:-Output
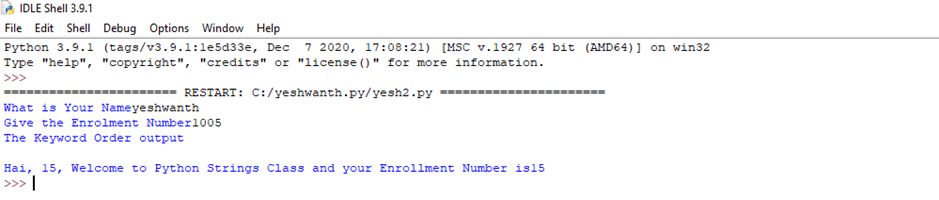
Example 4: Python string formatting using format() function-source code
a=20 b=10 sum=a+b sub=a-b print('The value of a is {} and b is {}'.format(a,b)) print('{2} is the sum of {0} and {1}'.format(a,b,sum)) print('{sub_value} is the subtraction of {value_a} and {value_b}'.format(value_a=a,value_b=b,sub_value=sub))
Example 4: Python string formatting using format() function-Output

Using %Operator
% – integer
% – float
% – string
% – hexadecimal
% – octal
Example 5: %Operator1- Source code
num=int(input("Enter a Value:")) add=num + 5 print("The sum is %d"%add)
Example 5: %Operator1- Output

Example 6: %Operator2- Source code
a=10 b=15 print("The Result of addition of %d+%d is %d"%(a,b,a+b))
Example 6: %Operator2- Output

Control Statements:
If statement:
If statement is the most simple decision-making statement. It is used to decide
Whether a certain statement or block of statement is executed or not i.e. if a condition is true then a block of statement is executed otherwise not.
Syntax:
If <condition>:
#Statements to execute if the condition is true
If condition:
Example 7: Python if Statement.-Source code
x=int(input("Give a Number:")) y=int(input("Give another Number:")) if(x>y): print("x = ",x,"is greater") if(x<y): print("y = ",y,"is greater")
Example 7: Python if Statement.-Output
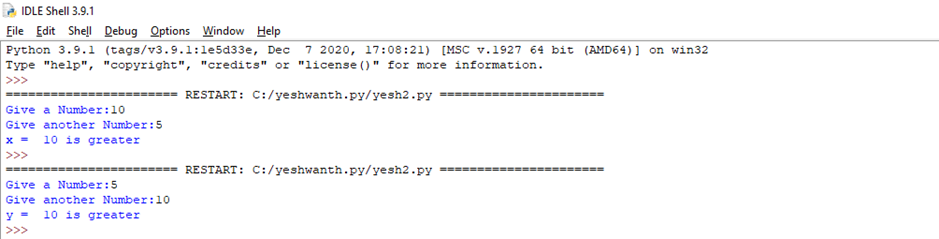
If-else Statement:
The simple if statement tells us that if condition is true it well execute a block of statements and if the condition is false it won’t.
Syntax:
If (condition):
# Executes this block if
# Condition is true
Else:
# Executes this block if
# Condition is false
Example8: Python if-else Statement-source code
x=int(input("Give a Number:")) y=int(input("Give a Another Number:")) if(x>y): print("x=",x,"is Greater") else: print("y=",y,"is Greater")
Example8: Python if-else Statement-Output
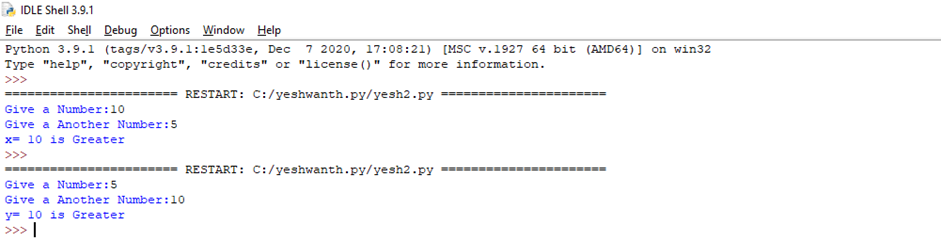
Nested-if Statement:
A nested if is an, if statement which is a part of another if block. i.e., we can place an if statement inside another if statement.
Syntax:
If (condition1):
# Executes when condition 1 is True
If (condition2):
# Executes when condition 2 is True
# if block2 is end here
# if block1 is end here
Another
If (condition1):
If (condition2):
# statement block1
else:
# statement block2
else:
If (condition3):
# statement block3
else:
# statement block
Example9: Python Nested if-Source code
x=int(input("Give a Number")) y=int(input("Give another Number")) z=int(input("Give another one")) if(x>y): if(x>z): print("x=",x,"is greatest") else: print("z=",z,"is greatest") else: if y>z: print("y=",y,"is greatest") else: print("z=",z,"is greatest")
Example9: Python Nested if-Output
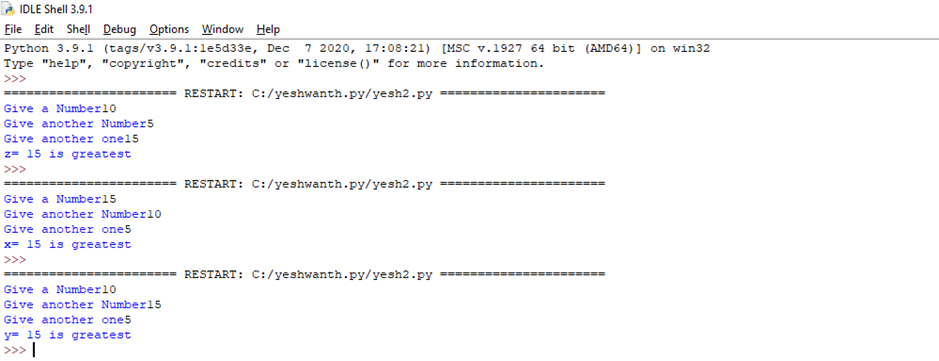
If-elif-else Statement or ladder if statement:
This is similar to we, deciding among form multiple options.
Syntax:
If (condition):
Statement
Elif(condition):
Statement
–
–
Else:
statement
Example10: If-elif-else Statement-Source code
i=int(input("Give the Number in the range of 10 to 20")) if(i==10): print("i is 10") elif(i==15): print("i is 15") elif(i==20): print("i is 20") else: print("i is not equal to any values present")
Example10:If-elif-else Statement-Output

Short Hand if Statement:
Syntax:
If condition: statement
Example 11: Python if shorthand-Source code
i=10 if i<15:print("i is less than 15")
Example 11: Python if shorthand-Output

Short hand if-else statement:
Syntax:
<statement_when_True>if<conditions>else<statement_when_False>
Example12: Python if else short hand-Source code
i=int(input("Give a Number ")) print(True) if i<15 else print(False)
Example12: Python if else short hand-Output
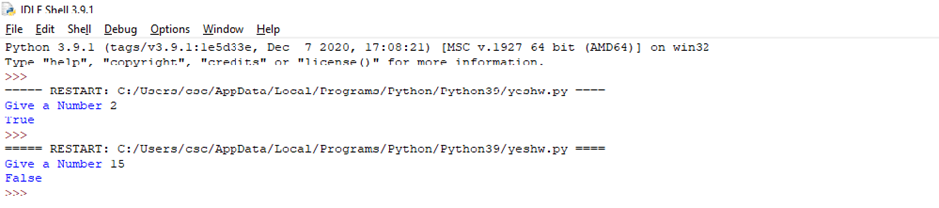
Chaining comparison operators in python:
Checking two or more conditions is very common in programming Languages.
Example13: Chaining comparison operators-Source code
x=5 print(1<x<10) print(10<x<20) print(x<10<x*10<100) print(10>x<=9) print(5 == x>4)
Example13: Chaining comparison operators-Output
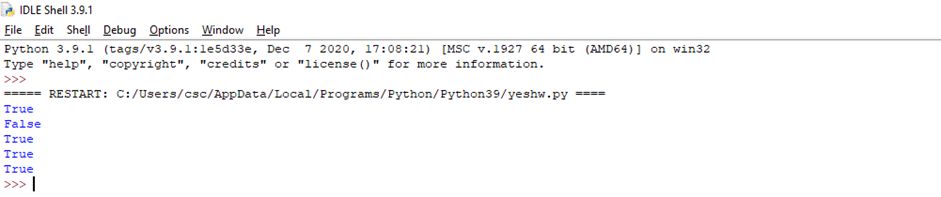
Loops in python:
While Loop:
In python, while loop is used to execute a block a block of statement repeatedly until the given condition is satisfied.
Syntax:
While expression:
Statement(s)
Example14:While Loop:-source code
count=0 while(count<3): count=count+1 print("Hi student")
Example14:While Loop:-Output
Using else statement with while loops:
Syntax:
While condition:
#execute these statements
Else:
#execute these statements
Example15:While Loop with else – Source code
count=0 while(count<3): count=count+1 print("Hi student") else: print("Bye")
Example15:While Loop with else – Output

For in loop:
Syntax:
For iterator_var in sequence:
statement(s)
It can be used to iterate over a range and also iterators.
Example16:For in loop – Source code
n=4 for i in range(0,n): print(i)
Example17:For in loop – Output

Example17:Python program to illustrate iterating over a list, tuple, string, dictionary, set.-Source code
print("List Iteration") l=["CSC","Computer","Education"] for i in l: print(i) print("\nTuple iteration") t=("CSC","Computer","Education") for i in t: print(i) print("\nString Iteraction") s="CSC Computer Education" for i in s: print(i) print("\nDictionary Iteration") d=dict() d['xyz']=123 d['abc']=345 for i in d: print("%s %d" %(i,d[i])) print("\nSet Iteration") set1={1,2,3,4} for i in set1: print(i),
Example17:Python program to illustrate iterating over a list, tuple, string, dictionary, set.-Output
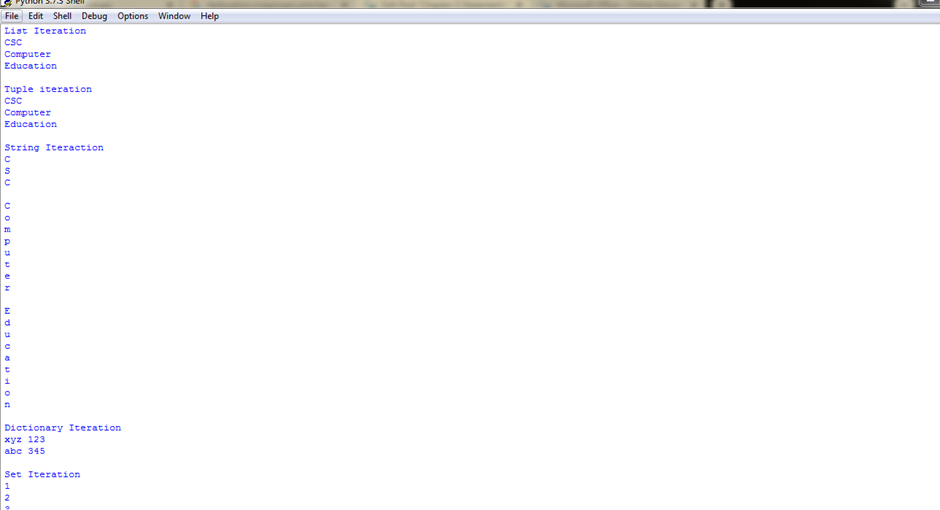
Iterating by the index of sequences:
Example18: Iterating by the index of sequences– Source code
Python program to illustrate iterating by index . list=["CSC","Computer","Education"] for index in range(len(list)): print(list[index])
Example18: Iterating by the index of sequences– Output

Using else statement with for loops:
Example19: Iterating by the index of sequences– Source code
#Python program to illustrate combining else with for list=["CSC","Computer","Education"] for index in range(len(list)): print(list[index]) else: print("Now inside Else Block")
Example19: Iterating by the index of sequences– Output

Nested Loop:
Python programming language allows to use one loop inside another loops.
Syntax:
For iterator_var in sequence:
For iterator_var in sequence:
Statements(s)
The syntax for a nested while loop statement in the python programming language is follows:
While expression:
While expression:
Statement(s)
Example20:Nested Loop – Source code
for i in range(1,5): for j in range(i): print(i,end=' ') print()
Example20:Nested Loop – Output

If we using print(j+1,end=’’) in the statement blok we got the output as
Example21: Source code
for i in range(1,5): for j in range(i): print(j+1,end=' ') print()
Example21: Output

Loop control Statements:
Continue statement:
It returns the control to the beginning of the loop, from the point from where it is executed thus skipping the statements below it in the loop.
Example22: Countinue satement-Source code
#Prints all letters except ‘C’ and ‘ ‘. for letter in 'CSC Computer Education': if letter == 'C' or letter == '': continue print('Current Letter:',letter) var = 10
Example22: Countinue satement-Output
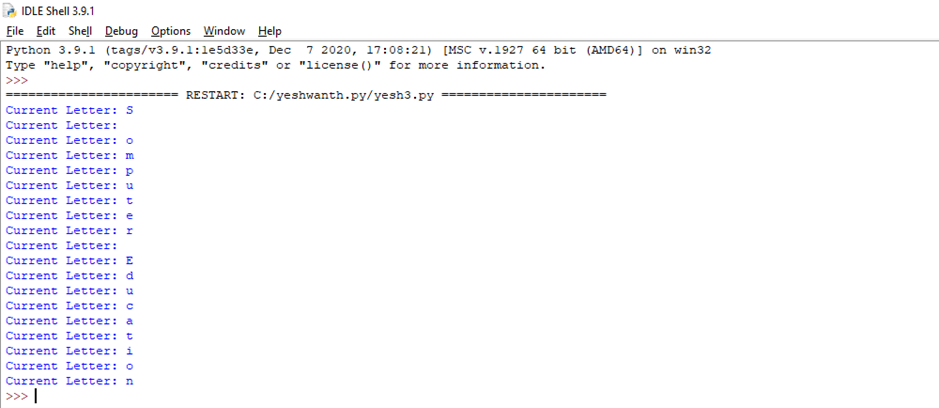
Break statement:
Unlike continue, this statement will shift the control to outside the loop and terminates it.
Example23: Break Statement – Source code
for letter in 'CSC Computer': if letter == 'o' or letter == 'e': break print('Current Letter:',letter)
Example23: Break Statement – Output
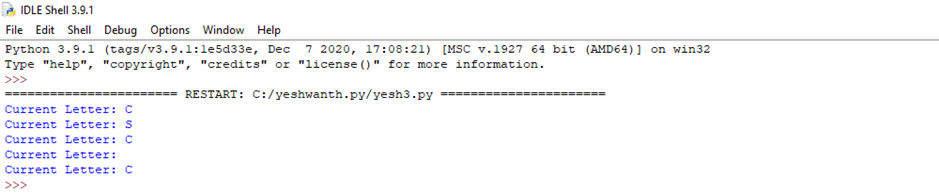
Pass Statement:
We use pass statement to write empty loops. Pass is also used for empty control statements, functions and classes. This is useful when we want to test run a set of statements before finishing it completely.
Example24: Pass Statement – Source code
#An empty loop for letter in 'CSC Computer': pass print('Last Letter:',letter)
Example24: Pass Statement – Output
