Python Mathematics
Mathematics involve Numbers and Operators that can be used on that. During initial stages, the main use of Computer Program were solving Complex Mathematical equations. These required the Numbers to be represented in different forms like Whole Numbers, Decimal point Numbers, Fractions and Complex numbers involving Real and Imaginary parts.
In Python, as we have already seen these Data Classes are available except Fractions. Fractions too can be used by importing fraction module. Few examples of Numeric Data
a = 524 b = 29645.32 c = 25 + 3j print ("Value of a is", a," and the data type is", type(a)) print ("Value of b is", b," and the data type is", type(b)) print ("Value of c is", c," and the data type is", type(c))
Output:

The Python Interpreter will automatically recognize the Class type of the Data that is being assigned.
Type Casting
Type Casting is nothing but conversion of one Data type to the other. There are 2. types of Type Casting available. One is Implicit Type Casting where the conversion is controlled by the Language itself. For example, when there are 2 types of Data in an expression, the smaller type of Data is converted to bigger one automatically.
d = b + a
29645.32 & 524 are added after converting the 524 as 524.0, this is done internally and the resultant value is saved in variable d, as float. Here int class is converted into float class, as float is bigger one than int.
print ("Value of d is", d, " and the data type is", type(d))
This will give the answer as 30169.32 and type as float. The other type casting is Explicit Type, where the conversion function are used by the programmer himself. int(), float(), complex() are the functions that can be used on the Number Data to convert from one type to another.
Here is the output of the above program:

Example
e=int (b) + a => 29645 + 524
Numbering Systems
There are 4 types of Numbering System in use. They are,
Name | Digit Count | Digits |
Decimal | 10 digits | 0-9 |
Binary | 2 digit | 0 and 1 |
Octal | 8 Digit | 0-7 |
Hexa Decimal | 16 Digit | 0-9, and A-F |
In Python all the Numbering Systems can be represented. But decimal numbering is default. Any Numeric Data is saved in Decimal form only. For representation in other forms we have to use the Functions available. Also if you want to provide Constants in other Numbering System the format is as below.
For Binary we have to use Ob or OB along with the number eg. 0b10001010
For Octal we have to use 00 or 00 along with the number eg. 0075261
For Hexa Decimal we have to use Ox or OX along with the number eg. 0X15A6E
The conversion functions to convert from one form to another are,
- bin() to convert to Binary number
- oct() to convert to Octal Number
- hex() to convert to Hexadecimal Number
Example
a = 4523 print("The Binary equivalent of", a," is", bin(a)); print("The Octal equivalent of", a, " is", oct(a)); print("The Hexa Decimal equivalent of", a, " is", hex(a));
Output:

For reverse, just use the variable name alone because the internal storage of any Numerical Data is always in Decimal system only.
Example
b=0B1000100001 print("The value in b is", b)
Output:
The value in b is 545
Python also allows mixed usage of Numbering system in Arithmetic Expression.
Example
c = 0x1AB92 + 0b01100011
Here the Binary number and Hexa-Decimal number will be converted into Decimal number and then processed. The Decimal equivalent of 0x1AB92 is 109458 and of 0b01100011 is 99. So these 2 will be added and saved in variable c.
0x1AB92+0b01100011 ==> 109458+99 = 109557
All these conversions are taken care by the language itself.
Floating point Arithmetic
Floating point Numbers Arithmetic is completely different in Python because of its internal storage precision.
1.1 + 2.2 is never equal to 3.3 in python, if ordinary Arithmetic expression is used. Are you wondering, How it can be True? If you don’t believe, check it out yourself in Python Shell window. Solution for this is to use the Decimal function defined in decimal Module of Python.
Example:
from decimal import Decimal as D a=D('1.1') b=D('2.2') c = D('3.3') print (a + b == c)
Output:

Here too we have to give the numeric values as String, otherwise even here we won’t get that as equal.
Another Interesting fact between / and // operators.
As already discussed, The Double Division operator in Python returns the floor value for both integer and floating-point arguments after division.
# A Python program to demonstrate use of “//” for both integers and floating points
print(5//2) print(-5//2) print(5.0//2)
Output:
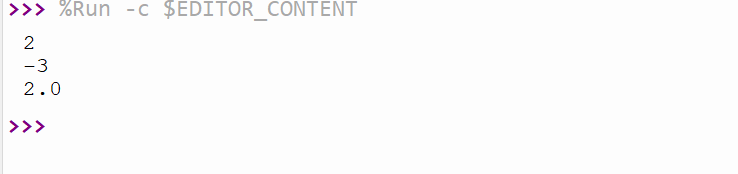
Floor means rounding off the value after decimal to its lower side. That’s why we get -3 for (-5//2), because in negative numbers -3 is less than -2.
The single division operator behaves normal when the values are of normal range. But it behaves abnormally for very large numbers. Consider the following example.
Examples 1:
#single division print(1000000002/2)
Output:
500000001.0
Try this,
print(int(((10**17) +2)/2))
Output:
50000000000000000
Where did that last 1 go, it should have been 50000000000000001. Confused, then try this,
print(((10**17) +2)//2)
Output:
50000000000000001
Yes, using ‘//’ operator on higher value numbers gives us correct Result while ‘/’ operator doesn’t give correct Result.
Example 2:
x=10000000000000000000006 if int(x/2) ==x // 2: print("Hello") else: print("World")
Output:
World
Again wondering, how did this happened? The Output should have been Hello if the single division operator behaved normally because 2 properly divides x. But the out-put is World because the results after Single Division Operator and Double Division Operator ARE NOT THE SAME.
This fact can be used for programs such as finding the sum of first n numbers for a large n.
n=10000000000 s1=int(n * (n + 1) / 2 ) s2=n^ * (n + 1) //2 print("Sum using single division operator: ", s1) print("Sum using double division operator: ", s2)
Output:
Sum using single division operator: 50000000005000003584
Sum using double division operator: 50000000005000000000
Thus the result found by using the single division operator is wrong, while the result found by using the double division operator is Correct. This is the huge benefit of Double Division Operator over Single Division Operator in Python.
For Mathematical Operations we can use these 3 Modules in Python
- Math
- Decimal
- Fractions
As in Arithmetic, we can use Fractional operations in Python, say for example like this,
a = 1/5 + 1/3
a = 8/15
For this we have to import fractions module.