Example 1 – CSS Backgrounds
<!DOCTYPE html> <head> <style> body{ background-color:pink; } div{ background-color: rgba(255,0,0,0.6)/*Blue color background with 30% opacity */ } body{ background-image:url("csclogo.jpg"); } body{ background-image:url("csclogo.jpg"); background-repeat: no-repeat; } /*Note: repeat=x, repeat-y are other repeating options.*/ body{ background-image: url("csclogo.jpg"); background-position: right top; background-repeat: no-repeat; } </style> </head> <body> <div>This is csc</div> </body> </html>
Example 1 – Output
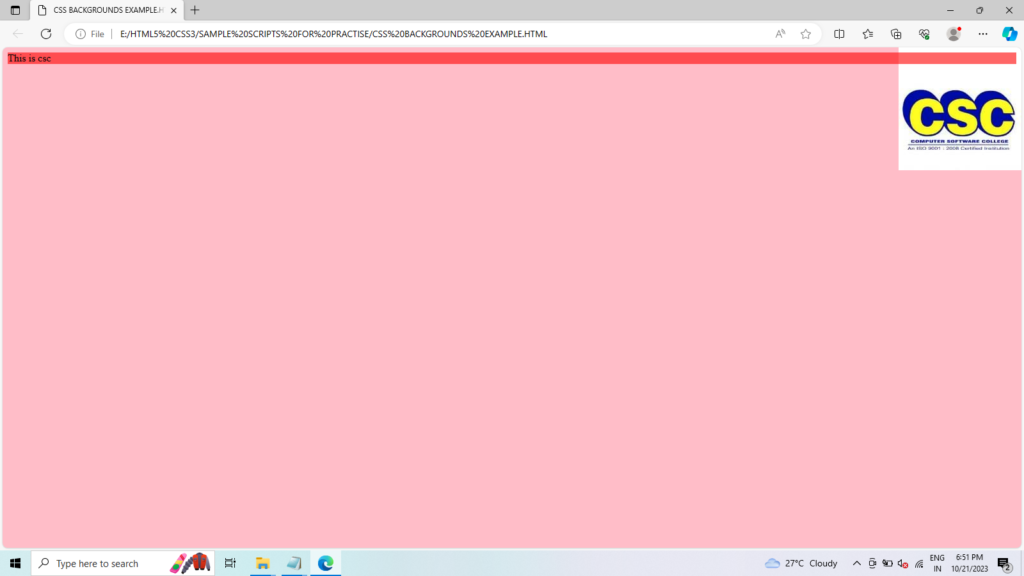
Example 2 – Internal CSS
<!doctype html> <html> <head> <style> h1 { color:green; text-decoration: underline; } p{ font-family: Trebuchet MS', sans-serif; font-size: 25px; } </style> </head> <body> <h1>Welcome to CSC Computer Education</h1> <p>HTML CSS</p> </body> </html>
Example 2 – Output
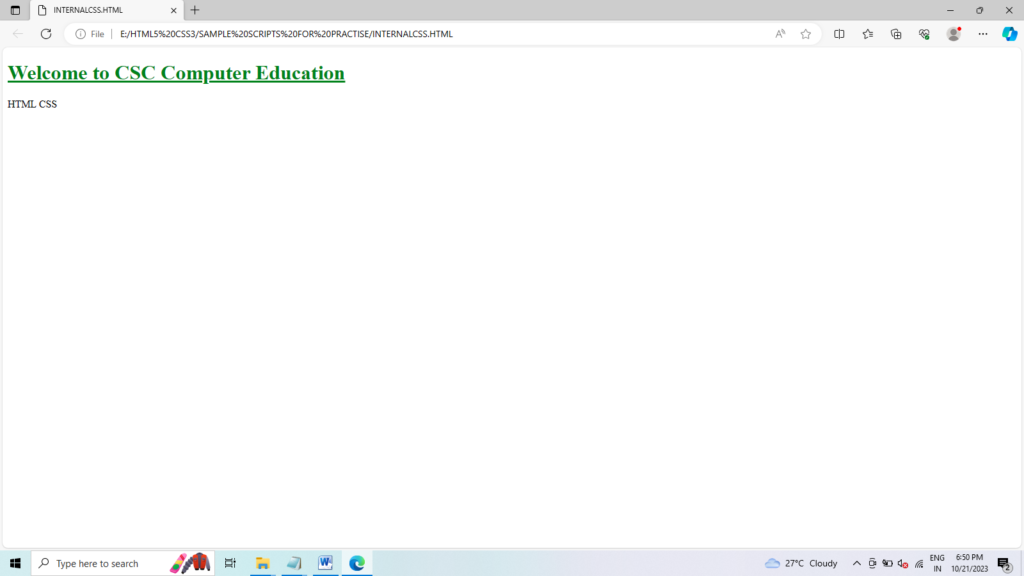
Example 3 – Inline CSS
<!doctype html> <html> <head> <title> Inline CSS </title> </head> <body> <h1 style="color: green; text-decoration: underline;">Welcome to CSC Computer Education</h1> <p style="font-family: Trebuchet MS', sans-serif; font-size: 25px;">HTML CSS</p> </body> </html>
Example 3 – Output
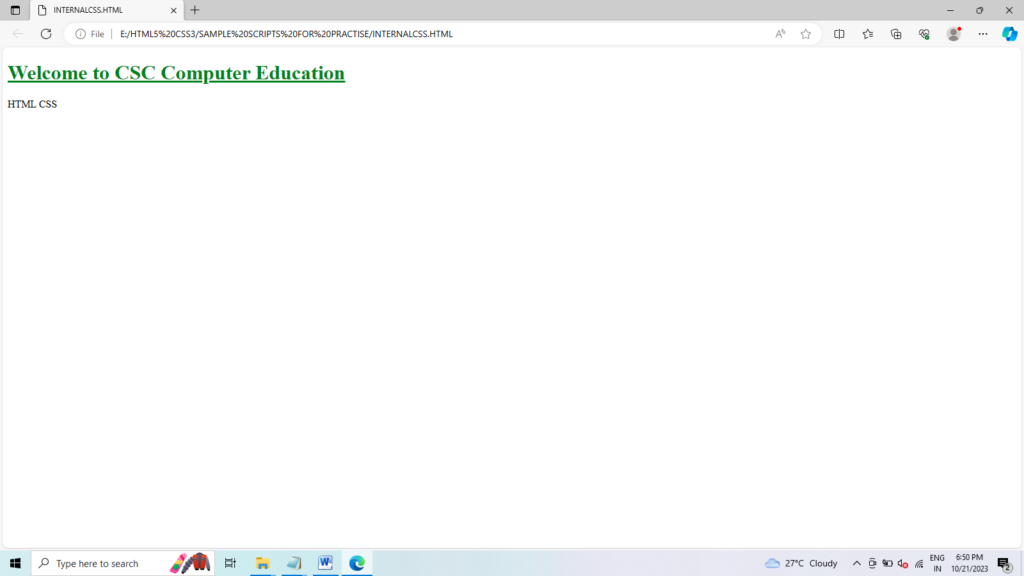
Example 4 – External CSS
External.html
<!DOCTYPE html> <head> <link rel="stylesheet" href="mystyle.css"> </head> <body> <h1>This is a heading</h1> <p>This is a paragraph.</p> </body> </html>
mystyle.css
body { background-color: lightblue; } h1 { color: navy; margin-left: 20px; }
Example 4 – Output
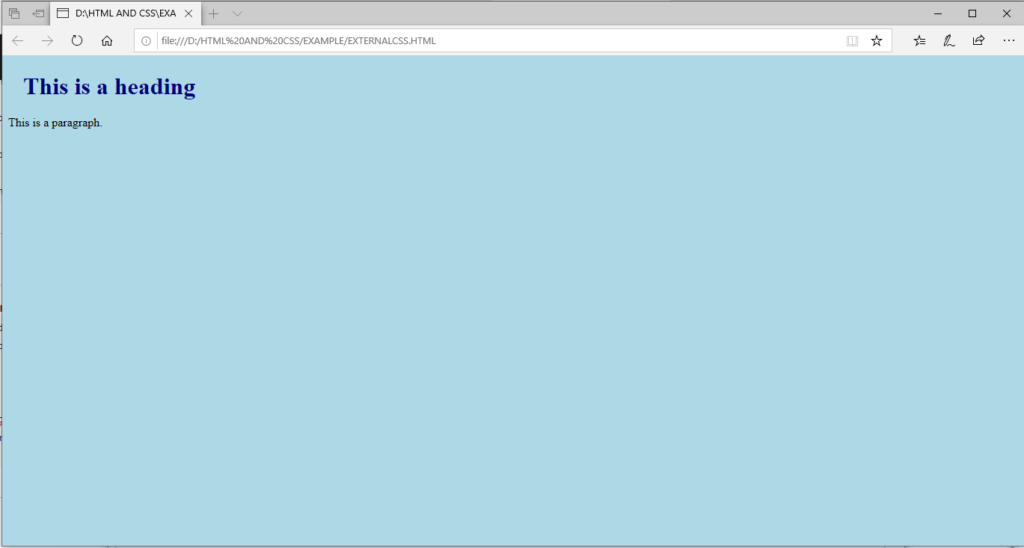
Example 5 – HTML figcaption Tag
<!doctype html> <html> <body> <img src="C:\Users\Admin\Pictures\wallpapper\csclogo.jpg" alt="CSC" /> <figure> <img src="C:\Users\Admin\Pictures\wallpapper\csclogo.jpg" alt="Student of the year" title="The BEST student of the year" /> <figcaption> Congrats!!! It's great to have you in our class. </figcaption> </figure> </body> </html>
Example 5 – Output
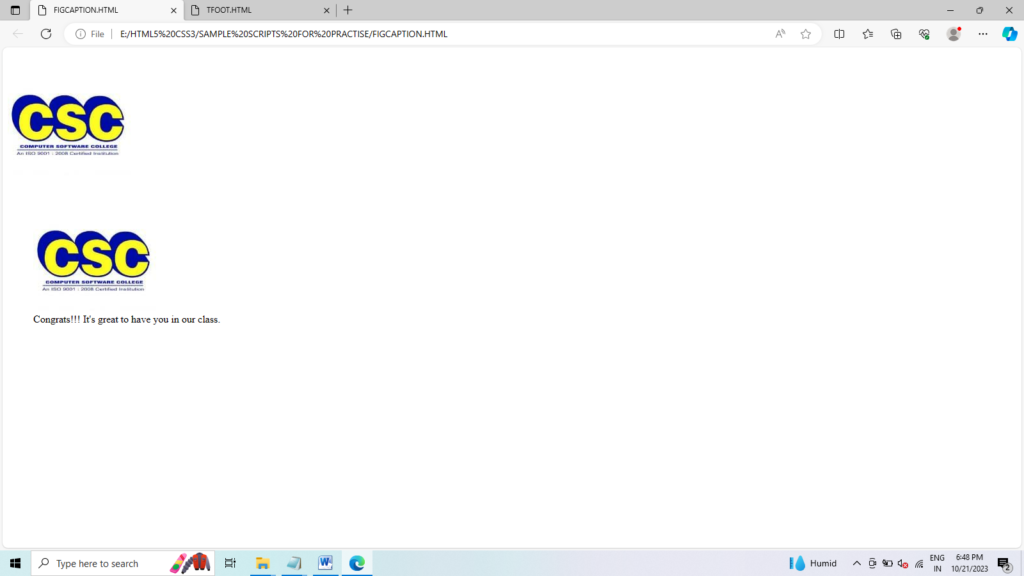
Example 6 – HTML5 Audio Tag
<audio controls> <source src="songname.mp3" type="audio/mp3"> <source src="songname.ogg" type="audio/ogg"> </audio>
Example 6 – Output
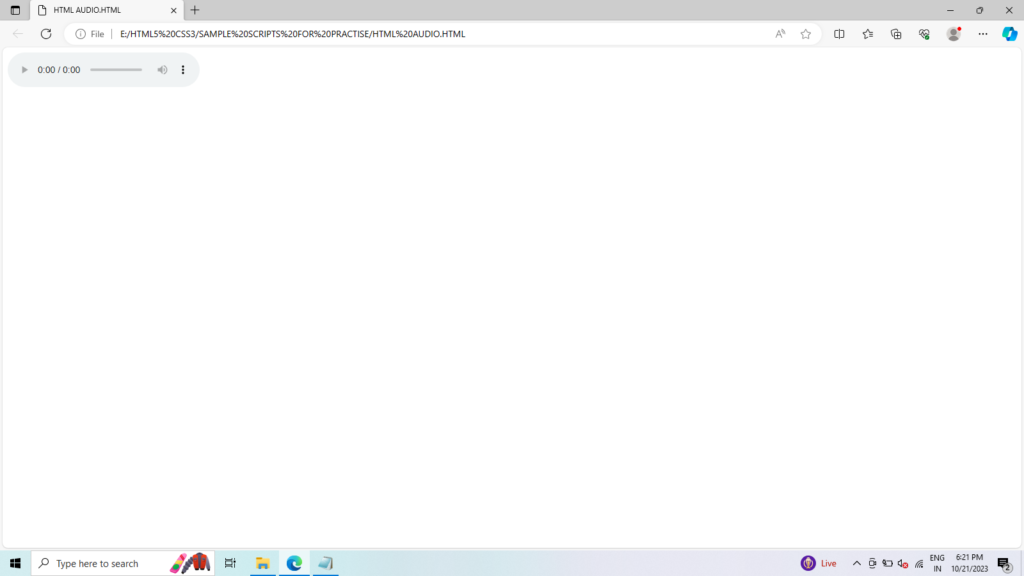
Example 7 – HTML5 Video Tag
<video controls> <source src="video.mp4" type="video/mp4"> <source src="video.ogg" type="video/ogg"> </video>
Example 7 – Output
Example 8 – HTML Table
<!doctype html> <html> <body> <table border="1"> <thead> <tr> <th>Season</th> <th>Months</th> <th>Max. Temperature<th> </tr> </thead> <tbody> <tr> <td>Summer</td> <td>March - June </td> <td>43°C</td> </tr> <tr> <td>Monsoon</td> <td>July-December</td> <td>30°C</td> </tr> <tr> <td>Winter</td> <td>January & February<td> <tfoot> <tr><td>welcome</td></tr></tfoot> </body> </html>
Example 8 – Output
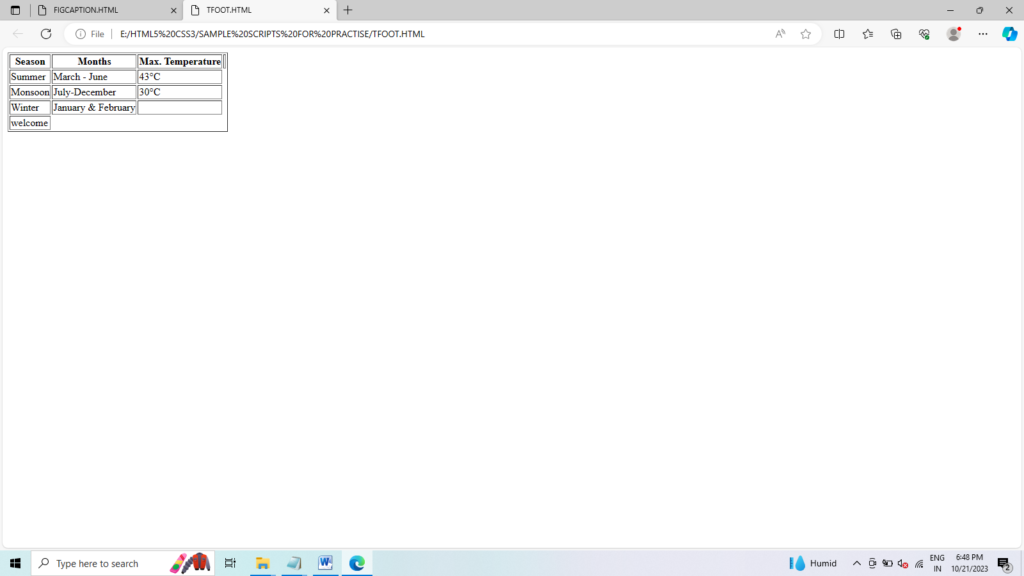