The General Form of a Class in Java…
Example 1 : BoxDemo
import java.io.*;
class Box{
double width;
double height;
double depth;
}
class BoxDemo{
public static void main(String args[])
{
Box mybox=new Box();
double vol;
mybox.width=10;
mybox.height=20;
mybox.depth=15;
vol=mybox.width*mybox.height*mybox.depth;
System.out.println("volume is "+vol);
}
}
import java.io.*;
class Box{
double width;
double height;
double depth;
}
class BoxDemo{
public static void main(String args[])
{
Box mybox=new Box();
double vol;
mybox.width=10;
mybox.height=20;
mybox.depth=15;
vol=mybox.width*mybox.height*mybox.depth;
System.out.println("volume is "+vol);
}
}
import java.io.*; class Box{ double width; double height; double depth; } class BoxDemo{ public static void main(String args[]) { Box mybox=new Box(); double vol; mybox.width=10; mybox.height=20; mybox.depth=15; vol=mybox.width*mybox.height*mybox.depth; System.out.println("volume is "+vol); } }

Example 2 : BoxDemo2
import java.io.*;
class Box{
double width;
double height;
double depth;
}
class BoxDemo2
{
public static void main(String args[])
{
Box mybox1=new Box();
Box mybox2=new Box();
double vol;
mybox1.width=10;
mybox1.height=20;
mybox1.depth=15;
mybox2.width=3;
mybox2.height=6;
mybox2.depth=9;
vol=mybox1.width*mybox1.height*mybox1.depth;
System.out.println("Volume is"+vol);
vol=mybox2.width*mybox2.height*mybox2.depth;
System.out.println("Volume is"+vol);
}
}
import java.io.*;
class Box{
double width;
double height;
double depth;
}
class BoxDemo2
{
public static void main(String args[])
{
Box mybox1=new Box();
Box mybox2=new Box();
double vol;
mybox1.width=10;
mybox1.height=20;
mybox1.depth=15;
mybox2.width=3;
mybox2.height=6;
mybox2.depth=9;
vol=mybox1.width*mybox1.height*mybox1.depth;
System.out.println("Volume is"+vol);
vol=mybox2.width*mybox2.height*mybox2.depth;
System.out.println("Volume is"+vol);
}
}
import java.io.*; class Box{ double width; double height; double depth; } class BoxDemo2 { public static void main(String args[]) { Box mybox1=new Box(); Box mybox2=new Box(); double vol; mybox1.width=10; mybox1.height=20; mybox1.depth=15; mybox2.width=3; mybox2.height=6; mybox2.depth=9; vol=mybox1.width*mybox1.height*mybox1.depth; System.out.println("Volume is"+vol); vol=mybox2.width*mybox2.height*mybox2.depth; System.out.println("Volume is"+vol); } }
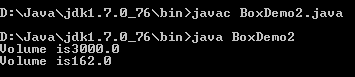
Assigning Object Reference Variables…
Example : ObjDemo
import java.io.*;
class ObjDemo
{
int a;
public static void main(String args[])
{
ObjDemo o1,o2;
o1=new ObjDemo();
o1.a=100;
o2=new ObjDemo();
o2.a=100;
if(o1==o2)
System.out.println("both are equal");
else
System.out.println("both are not equal");
o2=o1;
if(o1==o2)
System.out.println("both are equal");
else
System.out.println("both are not equal");
}
}
import java.io.*;
class ObjDemo
{
int a;
public static void main(String args[])
{
ObjDemo o1,o2;
o1=new ObjDemo();
o1.a=100;
o2=new ObjDemo();
o2.a=100;
if(o1==o2)
System.out.println("both are equal");
else
System.out.println("both are not equal");
o2=o1;
if(o1==o2)
System.out.println("both are equal");
else
System.out.println("both are not equal");
}
}
import java.io.*; class ObjDemo { int a; public static void main(String args[]) { ObjDemo o1,o2; o1=new ObjDemo(); o1.a=100; o2=new ObjDemo(); o2.a=100; if(o1==o2) System.out.println("both are equal"); else System.out.println("both are not equal"); o2=o1; if(o1==o2) System.out.println("both are equal"); else System.out.println("both are not equal"); } }
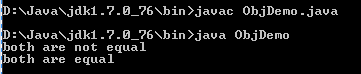